Create a simple spring boot application using gradle
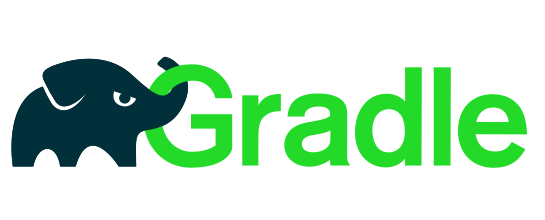
Overview
To create a simple spring boot application, as the very famous example “Hello World!”, you don’t need to use an IDE software. Fortunately, you can use only gradle CLI, and the Gradle website explains how to do this via its simple tutorial. I will try to sumarize it in this article
Getting Started
Initialize your project via gradle
gradle init --type java-application
It will create this directory structure adding some files
$ tree spring-hello-world/
spring-hello-world/
├── build.gradle
├── gradle
│ └── wrapper
│ ├── gradle-wrapper.jar
│ └── gradle-wrapper.properties
├── gradlew
├── gradlew.bat
├── settings.gradle
└── src
├── main
│ ├── java
│ │ └── spring
│ │ └── hello
│ │ └── world
│ │ └── App.java
│ └── resources
└── test
├── java
│ └── spring
│ └── hello
│ └── world
│ └── AppTest.java
└── resources
Modify the build.gradle
file adding the plugin and dependencies block
Creating a “Hello World” sample application
mkdir src/{main,test}/java/hello
Into src/main/java/hello
dir add two files: App.java
and HelloGradleController.java
And within the src/test/java/hello
create this file:
AppTest.java
Define the main class name for the spring boot jar file. In your build.gradle
file add this block
application {
mainClassName = 'hello.App'
}
bootJar {
mainClassName = 'hello.App'
}
At this point, you could build and run the application
./gradlew bootJar
Or using the jar file
java -jar ./build/libs/spring-hello-world.jar
Another way to run the Application is by executing the following Gradle command:
./gradlew bootRun
Sample: